SSRアプリケーションでのCookieベース認証トラブル解決法!Next.jsとExpressの連携実装ガイド
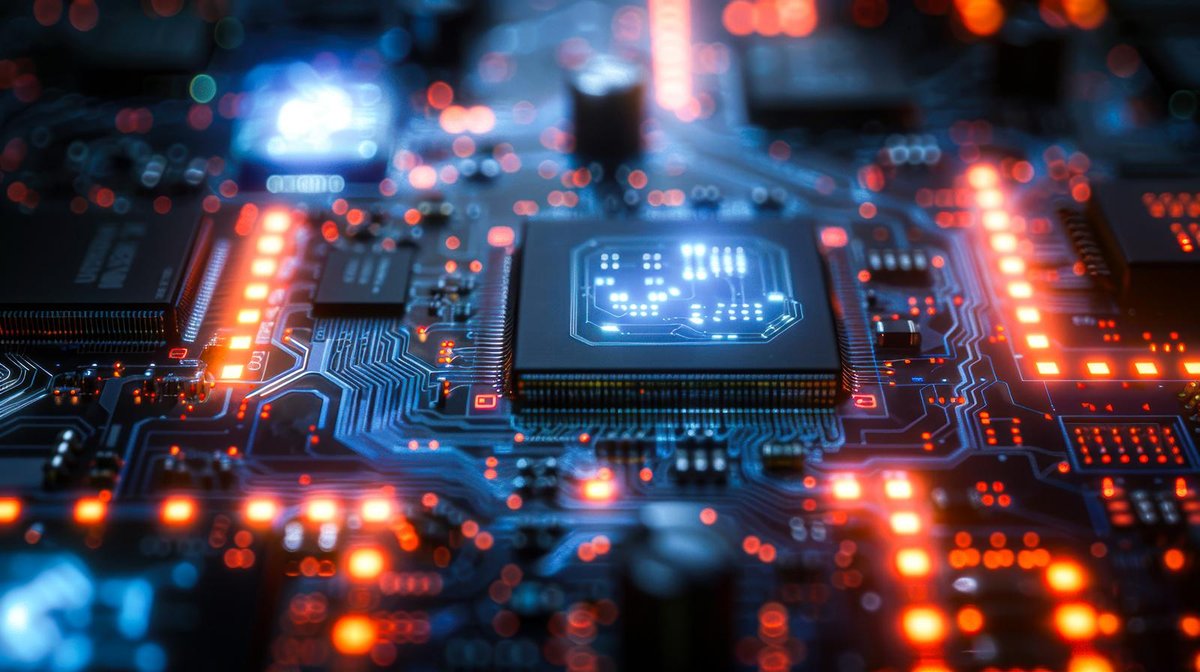
SSRアプリケーションの認証で直面する一般的な問題
Server-Side Rendering(SSR)アプリケーションでは、認証周りのトラブルに頭を悩ませることが少なくありません。特にCookieベースの認証を実装する場合、クライアントサイドとサーバーサイドの両方で正しく認証情報を処理する必要があり、様々な課題が生じます。
クライアントとサーバーの間でCookieが共有されない問題
SSRアプリケーションでよく遭遇する問題の一つが、ブラウザから送信されたCookieがサーバーサイドレンダリング時に正しく読み取れないというケースです。
// Next.jsのgetServerSidePropsでCookieが取得できない例
export async function getServerSideProps(context) {
console.log('Cookies from client:', context.req.cookies); // 空のオブジェクト or undefined
// APIリクエストを行うが、Cookieが送信されない
const response = await fetch('https://api.example.com/user', {
credentials: 'include' // これを指定しても解決しない場合がある
});
return {
props: {
user: null // 認証失敗
}
};
}
異なるドメイン間でのCookie送信の制限
フロントエンドとバックエンドAPIが異なるドメインやサブドメイン上にあると、ブラウザのSameSite Cookieポリシーによって、Cookieの送信が制限されることがあります。
// 正しく設定されていないCookieヘッダーの例
Set-Cookie: auth_token=abc123; Path=/; HttpOnly; SameSite=Strict
このようにSameSiteがStrictに設定されていると、別ドメインへのリクエスト時にCookieが送信されません。
開発環境と本番環境での挙動の違い
localhost環境では正常に動作するのに、本番環境ではCookieが送信されないというケースもよくあります。これは、開発環境ではhttpプロトコルを使用している一方、本番環境ではhttpsを使用しており、Secure属性の有無によってCookieの送信動作が変わるためです。
// 本番環境用のCookie設定例
Set-Cookie: auth_token=abc123; Path=/; HttpOnly; Secure; SameSite=None
サーバー側とクライアント側の状態の不一致
SSRでは、サーバーでレンダリングした時点の状態とクライアントでのハイドレーション後の状態が一致していないと、「ハイドレーションミスマッチ」エラーが発生することがあります。認証状態もその一つで、特にNext.jsのページ遷移時などに問題が顕在化します。
// クライアントとサーバーで異なる認証状態が発生する例
function ProfilePage({ serverSideUser }) {
const [clientSideUser, setClientSideUser] = useState(serverSideUser);
useEffect(() => {
// クライアントサイドで再度認証情報を取得
async function fetchUser() {
const res = await fetch('/api/user');
const user = await res.json();
setClientSideUser(user); // ここでサーバーサイドと異なる値になる可能性
}
fetchUser();
}, []);
// serverSideUserとclientSideUserが異なるとレンダリング結果も異なる
return <div>ユーザー名: {clientSideUser?.name || 'ゲスト'}</div>;
}
これらの問題を解決するためには、SSRの仕組みとCookieの扱い方を正しく理解し、フロントエンドとバックエンドの連携を適切に行う必要があります。次のセクションでは、Next.jsとExpressを使った具体的な実装方法を見ていきましょう。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
Next.jsとExpressで実装するCookieベース認証の基本
Next.jsフロントエンドとExpressバックエンドを使った安全なCookieベース認証の実装方法を見ていきましょう。SSRアプリケーションでの認証は、クライアントサイドのみの実装よりも複雑ですが、セキュリティや体験の向上につながります。
バックエンド(Express)側の実装
まず、Expressでの認証APIとCookie設定の基本的な実装例を示します。
// backend/server.js
const express = require('express');
const cookieParser = require('cookie-parser');
const jwt = require('jsonwebtoken');
const cors = require('cors');
const app = express();
const JWT_SECRET = process.env.JWT_SECRET || 'your-secret-key'; // 本番環境では必ず環境変数から
// ミドルウェアの設定
app.use(express.json());
app.use(cookieParser());
app.use(cors({
origin: process.env.NODE_ENV === 'production'
? 'https://your-frontend-domain.com'
: 'http://localhost:3000',
credentials: true // 重要:CORSリクエストでCookieを許可
}));
// ログインAPI
app.post('/api/login', (req, res) => {
const { username, password } = req.body;
// 実際のアプリではデータベースで検証
if (username === 'user' && password === 'password') {
// JWTトークンを生成
const token = jwt.sign(
{ userId: '123', username },
JWT_SECRET,
{ expiresIn: '1h' }
);
// Cookie設定(重要な部分)
res.cookie('auth_token', token, {
httpOnly: true, // JavaScriptからアクセス不可に
secure: process.env.NODE_ENV === 'production', // 本番環境ではHTTPSのみ
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
// 開発環境ではlax、本番クロスドメインではnone
maxAge: 60 * 60 * 1000, // 1時間
path: '/' // すべてのパスで利用可能に
});
res.json({ success: true, user: { id: '123', username } });
} else {
res.status(401).json({ success: false, message: '認証に失敗しました' });
}
});
// 認証状態確認API
app.get('/api/user', (req, res) => {
const token = req.cookies.auth_token;
if (!token) {
return res.status(401).json({ authenticated: false });
}
try {
// トークンの検証
const decoded = jwt.verify(token, JWT_SECRET);
res.json({
authenticated: true,
user: { id: decoded.userId, username: decoded.username }
});
} catch (error) {
// トークンの検証に失敗
res.status(401).json({ authenticated: false, error: error.message });
}
});
// ログアウトAPI
app.post('/api/logout', (req, res) => {
res.clearCookie('auth_token', {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
path: '/'
});
res.json({ success: true });
});
app.listen(4000, () => {
console.log('Server running on port 4000');
});
フロントエンド(Next.js)側の実装
次に、Next.jsフロントエンドでのCookieベース認証の基本実装です。
// frontend/lib/authService.js
// 認証APIを呼び出すサービス
export async function login(username, password) {
try {
const response = await fetch('http://localhost:4000/api/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ username, password }),
credentials: 'include' // 重要:Cookieを含める
});
return await response.json();
} catch (error) {
console.error('Login error:', error);
return { success: false, error: error.message };
}
}
export async function logout() {
try {
const response = await fetch('http://localhost:4000/api/logout', {
method: 'POST',
credentials: 'include' // 重要:Cookieを含める
});
return await response.json();
} catch (error) {
console.error('Logout error:', error);
return { success: false, error: error.message };
}
}
export async function getCurrentUser() {
try {
const response = await fetch('http://localhost:4000/api/user', {
credentials: 'include' // 重要:Cookieを含める
});
if (!response.ok) {
return { authenticated: false };
}
return await response.json();
} catch (error) {
console.error('Get user error:', error);
return { authenticated: false, error: error.message };
}
}
// frontend/pages/login.js
import { useState } from 'react';
import { useRouter } from 'next/router';
import { login } from '../lib/authService';
export default function Login() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [error, setError] = useState('');
const router = useRouter();
const handleSubmit = async (e) => {
e.preventDefault();
setError('');
const result = await login(username, password);
if (result.success) {
router.push('/dashboard'); // ログイン成功後のリダイレクト
} else {
setError(result.message || 'ログインに失敗しました');
}
};
return (
<div>
<h1>ログイン</h1>
{error && <div style={{ color: 'red' }}>{error}</div>}
<form onSubmit={handleSubmit}>
<div>
<label>
ユーザー名:
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
required
/>
</label>
</div>
<div>
<label>
パスワード:
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
required
/>
</label>
</div>
<button type="submit">ログイン</button>
</form>
</div>
);
}
SSRでの認証状態の取得
Next.jsでSSR時に認証状態を取得する実装例です。
// frontend/pages/dashboard.js
import { useEffect, useState } from 'react';
import { useRouter } from 'next/router';
import { getCurrentUser } from '../lib/authService';
// SSRでの認証チェック
export async function getServerSideProps(context) {
// サーバー側のCookieを取得して認証チェック
const { req, res } = context;
// SSRでのAPIリクエスト
// 注意: ブラウザと異なり、絶対URLが必要
const apiUrl = process.env.NODE_ENV === 'production'
? 'https://api.your-domain.com/api/user'
: 'http://localhost:4000/api/user';
const response = await fetch(apiUrl, {
headers: {
// ブラウザから送られてきたCookieをバックエンドに転送
Cookie: req.headers.cookie || ''
}
});
const data = await response.json();
// 認証されていない場合はログインページにリダイレクト
if (!data.authenticated) {
return {
redirect: {
destination: '/login',
permanent: false
}
};
}
// 認証済みの場合はユーザー情報を渡す
return {
props: {
user: data.user
}
};
}
export default function Dashboard({ user }) {
return (
<div>
<h1>ダッシュボード</h1>
<p>ようこそ、{user.username}さん!</p>
{/* ダッシュボードのコンテンツ */}
</div>
);
}
認証コンテキストの活用
効率的な実装のため、Reactコンテキストを使って認証状態を管理する方法も効果的です。
// frontend/context/AuthContext.js
import { createContext, useState, useEffect, useContext } from 'react';
import { getCurrentUser, login, logout } from '../lib/authService';
const AuthContext = createContext();
export function AuthProvider({ children }) {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
// コンポーネントマウント時に認証状態をチェック
async function loadUserFromCookie() {
try {
const data = await getCurrentUser();
if (data.authenticated) {
setUser(data.user);
} else {
setUser(null);
}
} catch (error) {
console.error('Failed to load user:', error);
setUser(null);
} finally {
setLoading(false);
}
}
loadUserFromCookie();
}, []);
const handleLogin = async (username, password) => {
const result = await login(username, password);
if (result.success) {
setUser(result.user);
}
return result;
};
const handleLogout = async () => {
const result = await logout();
if (result.success) {
setUser(null);
}
return result;
};
return (
<AuthContext.Provider
value={{
isAuthenticated: !!user,
user,
login: handleLogin,
logout: handleLogout,
loading
}}
>
{children}
</AuthContext.Provider>
);
}
export function useAuth() {
return useContext(AuthContext);
}
これらの基本実装は、シンプルなCookieベース認証の土台となります。しかし、実際の環境で動作させるには、開発環境と本番環境の違いを考慮した細かな調整が必要です。次のセクションでは、その違いを詳しく見ていきましょう。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
開発環境と本番環境での動作の違いを理解する
SSRアプリケーションでの認証実装において、開発環境(localhost)と本番環境の間には重要な違いがあります。これらの違いを理解せずに実装すると、「開発環境では動くのに本番では動かない」という状況に陥りがちです。
セキュリティ制約の違い
プロトコルの違い(HTTP vs HTTPS)
開発環境ではHTTPを使用することが多いですが、本番環境ではHTTPSを使用するのが一般的です。これによって以下のような違いが生じます:
// 開発環境でのCookie設定
res.cookie('auth_token', token, {
httpOnly: true,
secure: false, // HTTP接続でも送信可能
sameSite: 'lax'
});
// 本番環境でのCookie設定
res.cookie('auth_token', token, {
httpOnly: true,
secure: true, // HTTPSでのみ送信される
sameSite: 'none' // クロスサイトリクエストを許可
});
特にsecure
属性の違いにより、本番環境ではHTTPS接続でなければCookieが送信されなくなります。
SameSite属性の影響
ブラウザはSameSite Cookieポリシーにより、異なるドメインへのリクエスト時のCookie送信を制限しています。開発環境と本番環境で異なる設定が必要です:
// 環境に応じたCookie設定
const cookieOptions = {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
maxAge: 60 * 60 * 1000 // 1時間
};
res.cookie('auth_token', token, cookieOptions);
環境 | SameSite設定 | 理由 |
---|---|---|
開発環境 | 'lax' | 同一ドメイン内(localhost)での動作が主で、厳しい制限は不要 |
本番環境 | 'none' | フロントエンドとAPIが異なるドメインの場合、クロスサイトCookieの送信が必要 |
注意点として、'none'を指定する場合は必ずsecure: true
と組み合わせる必要があります。そうしないと最新のブラウザはCookieを拒否します。
ドメイン設定の違い
開発環境ではlocalhostを使用しますが、本番環境では実際のドメインを使用します。これにより、CORS設定やCookieのドメイン属性の設定が変わってきます。
// 環境に応じたCORSとCookie設定
const corsOptions = {
origin: process.env.NODE_ENV === 'production'
? 'https://your-frontend-domain.com'
: 'http://localhost:3000',
credentials: true
};
const cookieOptions = {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
domain: process.env.NODE_ENV === 'production'
? '.your-domain.com' // サブドメイン間でCookieを共有したい場合
: undefined // localhost用
};
Next.jsのSSRにおける環境差異
Next.jsのSSRモードでは、サーバー側からのAPI呼び出しに関しても環境の違いに注意が必要です。
// Next.jsのgetServerSidePropsでの環境対応
export async function getServerSideProps(context) {
const { req } = context;
// 環境に応じたAPIのベースURL
const API_BASE_URL = process.env.NODE_ENV === 'production'
? 'https://api.your-domain.com'
: 'http://localhost:4000';
try {
const response = await fetch(`${API_BASE_URL}/api/user`, {
headers: {
Cookie: req.headers.cookie || '' // ブラウザから送られてきたCookieを転送
}
});
const data = await response.json();
// データ処理と返却
return {
props: {
user: data.user || null,
isAuthenticated: !!data.authenticated
}
};
} catch (error) {
console.error('API call error:', error);
return {
props: {
user: null,
isAuthenticated: false
}
};
}
}
環境差異に対応するための実装パターン
環境の違いに対応するための実装パターンをいくつか紹介します。
環境変数を活用した設定
Next.jsでは.env.development
と.env.production
のようにファイルを分けて環境ごとの変数を管理できます。
# .env.development
NEXT_PUBLIC_API_URL=http://localhost:4000
COOKIE_SECURE=false
COOKIE_SAME_SITE=lax
# .env.production
NEXT_PUBLIC_API_URL=https://api.your-domain.com
COOKIE_SECURE=true
COOKIE_SAME_SITE=none
これをコードで利用:
// 環境変数に基づく設定
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
const cookieOptions = {
httpOnly: true,
secure: process.env.COOKIE_SECURE === 'true',
sameSite: process.env.COOKIE_SAME_SITE,
maxAge: 60 * 60 * 1000
};
開発環境専用のプロキシの活用
開発環境でのCORS問題を回避するため、Next.jsのAPI Routesを使ってプロキシを実装する方法もあります。
// pages/api/proxy/[...path].js
export default async function handler(req, res) {
const { path } = req.query;
const apiUrl = `http://localhost:4000/${path.join('/')}`;
try {
const response = await fetch(apiUrl, {
method: req.method,
headers: {
'Content-Type': 'application/json',
Cookie: req.headers.cookie || ''
},
body: req.method !== 'GET' ? JSON.stringify(req.body) : undefined
});
// レスポンスヘッダーの転送
const cookies = response.headers.get('set-cookie');
if (cookies) {
res.setHeader('Set-Cookie', cookies);
}
const data = await response.json();
res.status(response.status).json(data);
} catch (error) {
res.status(500).json({ error: error.message });
}
}
このプロキシを使用すれば、フロントエンドからは同一ドメインへのリクエストになるため、開発環境でもCORS問題を回避できます。
条件付きリクエスト設定
APIリクエストの設定を環境によって動的に変更する方法も有効です。
// auth.js
export async function makeAuthenticatedRequest(url, options = {}) {
const isProduction = process.env.NODE_ENV === 'production';
const baseUrl = isProduction
? 'https://api.your-domain.com'
: 'http://localhost:4000';
const fetchOptions = {
...options,
credentials: 'include', // Cookieを含める
headers: {
...options.headers,
'Content-Type': 'application/json'
}
};
try {
const response = await fetch(`${baseUrl}${url}`, fetchOptions);
return await response.json();
} catch (error) {
console.error('API request failed:', error);
return { error: error.message };
}
}
これらのパターンを活用することで、開発環境と本番環境の両方で一貫して動作する認証システムを構築できます。次のセクションでは、CORSの正しい設定方法について詳しく見ていきましょう。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
Cross-Origin Resource Sharing (CORS)の正しい設定方法
SSRアプリケーションでのCookieベース認証の最大の障壁の一つが、CORS(Cross-Origin Resource Sharing)の設定です。特にフロントエンドとバックエンドが異なるドメインにある場合、適切なCORS設定なしではCookieがうまく送受信できません。
CORSとは何か
CORSは、あるオリジン(ドメイン、プロトコル、ポートの組み合わせ)から別のオリジンのリソースへのアクセスを制御するためのセキュリティメカニズムです。ブラウザはセキュリティ上の理由で、JavaScriptからの異なるオリジンへのリクエストを制限します。
// オリジンの例
https://frontend.example.com // プロトコル: https, ドメイン: frontend.example.com, ポート: 443(デフォルト)
http://localhost:3000 // プロトコル: http, ドメイン: localhost, ポート: 3000
同一オリジンポリシーにより、異なるオリジンへのリクエストは制限されますが、CORSを設定することでこの制限を緩和することができます。
ExpressバックエンドでのCORS設定
Node.js/ExpressバックエンドでのCORS設定には、cors
ミドルウェアを使用するのが一般的です。ただし、Cookieをやり取りする場合は特別な設定が必要です。
const express = require('express');
const cors = require('cors');
const app = express();
// 適切なCORS設定
app.use(cors({
origin: process.env.NODE_ENV === 'production'
? 'https://your-frontend-domain.com' // 本番環境のフロントエンドドメイン
: 'http://localhost:3000', // 開発環境のフロントエンドドメイン
credentials: true, // 重要:Cookieを含むリクエストを許可
methods: ['GET', 'POST', 'PUT', 'DELETE', 'OPTIONS'], // 許可するHTTPメソッド
allowedHeaders: ['Content-Type', 'Authorization'] // 許可するヘッダー
}));
// 他のミドルウェアや設定...
credentials: true
は特に重要です。これにより、クロスオリジンリクエストにもCookieを含めることが許可されます。
注意すべき重要な点
originの設定:
*
(ワイルドカード)の代わりに、具体的なドメインを指定する必要があります。credentials: true
とorigin: '*'
は併用できません。// 間違った設定 - credentials: trueと併用できない app.use(cors({ origin: '*', credentials: true })); // 正しい設定 - 具体的なドメインを指定 app.use(cors({ origin: 'https://your-frontend-domain.com', credentials: true }));
複数のオリジンを許可する場合: 関数を使って動的に判断できます。
const allowedOrigins = [ 'https://your-frontend-domain.com', 'https://staging.your-domain.com', 'http://localhost:3000' ]; app.use(cors({ origin: function(origin, callback) { // origin がnullの場合はサーバー内部からのリクエスト if (!origin || allowedOrigins.indexOf(origin) !== -1) { callback(null, true); } else { callback(new Error('CORS policy violation')); } }, credentials: true }));
Access-Control-Allow-Originヘッダー:
credentials: true
を設定すると、ブラウザはAccess-Control-Allow-Origin
ヘッダーが具体的な値(*
でない)であることを要求します。
Next.jsフロントエンドの設定
Next.jsのフロントエンドからバックエンドAPIにリクエストを送る際には、credentials: 'include'
を指定する必要があります。
// Frontend API client
async function fetchFromApi(url, options = {}) {
const response = await fetch(`https://api.your-domain.com${url}`, {
...options,
credentials: 'include', // 重要:Cookieをリクエストに含める
headers: {
'Content-Type': 'application/json',
...options.headers
}
});
return await response.json();
}
また、Next.jsのgetServerSideProps
やgetStaticProps
内でのAPI呼び出しでは、オリジン間でCookieを送信するために追加の設定が必要です。
export async function getServerSideProps(context) {
const { req } = context;
try {
const response = await fetch('https://api.your-domain.com/user', {
headers: {
Cookie: req.headers.cookie || '' // 重要:ブラウザからのCookieを転送
}
});
// 以下データ処理...
} catch (error) {
// エラーハンドリング...
}
}
Next.jsのAPI Routesを使ったプロキシ
Next.jsアプリケーションでCORSを回避する一つの方法は、Next.jsのAPI Routesをプロキシとして使用することです。これにより、フロントエンド(ブラウザ)からのリクエストは常に同一オリジンとなり、CORSの問題が発生しません。
// pages/api/auth/[...path].js
export default async function handler(req, res) {
const { path } = req.query;
const apiUrl = `https://api.your-domain.com/${path.join('/')}`;
try {
const response = await fetch(apiUrl, {
method: req.method,
headers: {
'Content-Type': 'application/json',
Cookie: req.headers.cookie || ''
},
body: req.method !== 'GET' && req.method !== 'HEAD'
? JSON.stringify(req.body)
: undefined
});
// Set-Cookie ヘッダーの転送
const setCookieHeader = response.headers.get('set-cookie');
if (setCookieHeader) {
res.setHeader('Set-Cookie', setCookieHeader);
}
// レスポンスデータの転送
const data = await response.json();
res.status(response.status).json(data);
} catch (error) {
console.error('API proxy error:', error);
res.status(500).json({ error: 'Internal Server Error' });
}
}
このプロキシを使うと、フロントエンドからのコードは次のようになります:
// 同一オリジンへのリクエストになるのでCORSの問題が起きない
const response = await fetch('/api/auth/login', {
method: 'POST',
credentials: 'same-origin', // same-originでも動作する
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ username, password })
});
よくあるCORS関連エラーとその解決法
1. "No 'Access-Control-Allow-Origin' header is present"
このエラーは、サーバーが適切なCORSヘッダーを返していない場合に発生します。
解決策:
- バックエンドで
cors
ミドルウェアが正しく設定されているか確認 origin
の値がリクエスト元のオリジンと一致しているか確認
// 修正例
app.use(cors({
origin: 'http://localhost:3000', // リクエスト元のオリジンに合わせる
credentials: true
}));
2. "The value of the 'Access-Control-Allow-Origin' header in the response must not be the wildcard '*' when the request's credentials mode is 'include'"
このエラーは、credentials: 'include'
でリクエストを送っているのに、サーバー側でorigin: '*'
を設定している場合に発生します。
解決策:
- サーバー側の
origin
設定を具体的なドメインに変更
// 修正例
app.use(cors({
origin: 'http://localhost:3000', // ワイルドカード '*' ではなく具体的なドメイン
credentials: true
}));
3. "Response to preflight request doesn't pass access control check"
このエラーは、プリフライトリクエスト(OPTIONSメソッド)の処理が不適切な場合に発生します。
解決策:
methods
に必要なHTTPメソッドが含まれているか確認- カスタムヘッダーを使用している場合は
allowedHeaders
に含める
// 修正例
app.use(cors({
origin: 'http://localhost:3000',
credentials: true,
methods: ['GET', 'POST', 'PUT', 'DELETE', 'OPTIONS'],
allowedHeaders: ['Content-Type', 'Authorization', 'X-Custom-Header']
}));
本番環境のセキュリティ強化
本番環境でのCORS設定は、セキュリティと使いやすさのバランスを取ることが重要です。特に、認証情報を含むリクエストでは慎重な設定が必要です。
// 本番環境向けの安全なCORS設定
const isProduction = process.env.NODE_ENV === 'production';
const allowedOrigins = isProduction
? ['https://your-frontend-domain.com', 'https://admin.your-domain.com']
: ['http://localhost:3000'];
app.use(cors({
origin: function(origin, callback) {
// Server-to-server リクエスト(originがnull)を許可
if (!origin || allowedOrigins.includes(origin)) {
callback(null, true);
} else {
callback(new Error(`Origin ${origin} not allowed by CORS`));
}
},
credentials: true,
methods: ['GET', 'POST', 'PUT', 'DELETE', 'OPTIONS'],
allowedHeaders: ['Content-Type', 'Authorization'],
// プリフライトリクエストのキャッシュ時間(秒)
maxAge: 86400 // 24時間
}));
これらの設定を正しく行うことで、異なるドメイン間でもCookieベースの認証が正常に機能するようになります。特に、SSRアプリケーションでは、フロントエンドサーバーとAPIサーバー間の通信が安全かつ適切に行われることが重要です。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
HTTPOnly Cookieを活用したセキュアな実装テクニック
SSRアプリケーションでCookieベースの認証を実装する際、セキュリティは最も重要な考慮事項の一つです。特に、HTTPOnly Cookieを使用することで、クライアントサイドのJavaScriptからのCookie操作リスクを大幅に軽減できます。
HTTPOnly Cookieとは
HTTPOnly Cookieは、JavaScriptからアクセスできないように設定されたCookieです。通常のCookieはdocument.cookie
を通じてJavaScriptからアクセス・変更が可能ですが、HTTPOnly属性が設定されたCookieはHTTPリクエスト時にのみブラウザによって送信され、JavaScriptからは見えなくなります。
// HTTPOnly属性の設定
res.cookie('auth_token', token, {
httpOnly: true, // JavaScriptからアクセス不可に
// 他の属性...
});
JWTとの組み合わせによるセキュアな認証トークン管理
認証トークンとしてJWT(JSON Web Token)を使用し、それをHTTPOnly Cookieとして保存する方法が、現代のSSRアプリケーションでは一般的です。
// サーバーサイド(Express)でのJWT生成とCookie設定
const jwt = require('jsonwebtoken');
const JWT_SECRET = process.env.JWT_SECRET || 'development-secret-key';
app.post('/api/login', (req, res) => {
// ユーザー認証ロジック...
// JWT生成
const token = jwt.sign(
{ userId: user.id, username: user.username },
JWT_SECRET,
{ expiresIn: '1h' }
);
// HTTPOnly Cookieとして設定
res.cookie('auth_token', token, {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
maxAge: 60 * 60 * 1000 // 1時間
});
// レスポンス(トークンそのものは含めない)
res.json({
success: true,
user: {
id: user.id,
username: user.username
// パスワードなどの機密情報は含めない
}
});
});
セキュリティを高めるために設定すべきCookie属性
HTTPOnly以外にも、Cookieのセキュリティを高めるための属性があります。
// セキュアなCookie設定の例
const cookieOptions = {
httpOnly: true, // JavaScriptからアクセス不可
secure: true, // HTTPS経由でのみ送信
sameSite: 'strict', // 同一サイトからのリクエストのみCookieを送信
maxAge: 60 * 60 * 1000, // 有効期限(ミリ秒)
path: '/', // Cookieが有効なパス
domain: 'example.com', // Cookieが有効なドメイン(サブドメイン含む場合)
};
各属性の効果:
属性 | 効果 | 推奨設定 |
---|---|---|
httpOnly | JavaScriptからのアクセスを防止 | true |
secure | HTTPS接続でのみCookieを送信 | 本番環境ではtrue |
sameSite | クロスサイトリクエストでのCookie送信を制限 | 同一ドメイン内ならstrict、異なるドメイン間ならnone(secureと併用) |
maxAge | Cookieの有効期限 | JWT有効期限と一致させる |
path | Cookieが有効なパス | 通常は'/' |
domain | Cookieが有効なドメイン | サブドメイン間で共有する場合のみ設定 |
XSS攻撃からの保護
HTTPOnly Cookieを使用しても、クロスサイトスクリプティング(XSS)攻撃に対する完全な保護にはなりません。追加の対策が必要です。
// Expressでのセキュリティヘッダー設定
const helmet = require('helmet');
app.use(helmet()); // 各種セキュリティヘッダーを設定
// または個別に設定
app.use(helmet.contentSecurityPolicy()); // Content-Security-Policy
app.use(helmet.xssFilter()); // X-XSS-Protection
app.use(helmet.noSniff()); // X-Content-Type-Options
Next.jsでの実装:
// next.config.js
module.exports = {
async headers() {
return [
{
source: '/(.*)',
headers: [
{
key: 'Content-Security-Policy',
value: "default-src 'self'; script-src 'self' 'unsafe-inline';"
},
{
key: 'X-XSS-Protection',
value: '1; mode=block'
},
{
key: 'X-Content-Type-Options',
value: 'nosniff'
}
]
}
];
}
};
CSRF対策
HTTPOnly Cookieを使用していても、CSRF(クロスサイトリクエストフォージェリ)攻撃のリスクは残ります。これを軽減するための対策が必要です。
// Expressでのcsrf対策
const csrf = require('csurf');
const csrfProtection = csrf({
cookie: {
httpOnly: true,
secure: process.env.NODE_ENV === 'production'
}
});
// CSRF保護が必要なルートで使用
app.post('/api/change-password', csrfProtection, (req, res) => {
// CSRFトークンが検証され、有効な場合のみ実行される
});
// CSRFトークンの提供
app.get('/api/csrf-token', csrfProtection, (req, res) => {
res.json({ csrfToken: req.csrfToken() });
});
フロントエンド側での使用:
// CSRFトークンを取得して使用
function PasswordChangeForm() {
const [csrfToken, setCsrfToken] = useState('');
useEffect(() => {
// CSRFトークンの取得
async function fetchCsrfToken() {
const response = await fetch('/api/csrf-token', {
credentials: 'include' // Cookieを含める
});
const data = await response.json();
setCsrfToken(data.csrfToken);
}
fetchCsrfToken();
}, []);
const handleSubmit = async (e) => {
e.preventDefault();
// フォームデータの送信
const response = await fetch('/api/change-password', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'CSRF-Token': csrfToken // CSRFトークンをヘッダーに含める
},
credentials: 'include',
body: JSON.stringify({
newPassword: 'new-password'
})
});
// レスポンス処理...
};
return (
<form onSubmit={handleSubmit}>
{/* フォームの内容 */}
<input type="hidden" name="_csrf" value={csrfToken} />
<button type="submit">パスワード変更</button>
</form>
);
}
ダブルサブミット Cookie パターン
CSRFミドルウェアを使用せずに、より単純な「ダブルサブミットCookieパターン」を実装することもできます。
// サーバー側
app.post('/api/login', (req, res) => {
// 認証ロジック...
// セキュアトークンの生成
const csrfToken = crypto.randomBytes(16).toString('hex');
// AuthトークンをHttpOnly Cookieとして設定
res.cookie('auth_token', jwtToken, {
httpOnly: true,
secure: true
});
// CSRFトークンを非HttpOnly Cookieとして設定(JavaScriptからアクセス可能)
res.cookie('csrf_token', csrfToken, {
httpOnly: false,
secure: true
});
res.json({
success: true,
user: { /* ユーザー情報 */ }
});
});
// 保護されたエンドポイント
app.post('/api/sensitive-action', (req, res) => {
const csrfTokenCookie = req.cookies.csrf_token;
const csrfTokenHeader = req.headers['x-csrf-token'];
// トークンの比較
if (!csrfTokenCookie || !csrfTokenHeader || csrfTokenCookie !== csrfTokenHeader) {
return res.status(403).json({ error: 'CSRF検証失敗' });
}
// 正当なリクエストとして処理
});
フロントエンド側:
// リクエスト送信
function submitSensitiveAction() {
// CookieからCSRFトークンを読み取る
const csrfToken = getCookie('csrf_token');
fetch('/api/sensitive-action', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-CSRF-Token': csrfToken
},
credentials: 'include',
body: JSON.stringify({ /* データ */ })
});
}
// Cookieを取得するヘルパー関数
function getCookie(name) {
const value = `; ${document.cookie}`;
const parts = value.split(`; ${name}=`);
if (parts.length === 2) return parts.pop().split(';').shift();
}
リフレッシュトークンパターンの実装
短期間のアクセストークンと長期間のリフレッシュトークンを使い分けるパターンも、セキュリティを高める効果的な方法です。
// ログイン時にアクセストークンとリフレッシュトークンを発行
app.post('/api/login', (req, res) => {
// 認証ロジック...
// 短期間のアクセストークン(15分)
const accessToken = jwt.sign(
{ userId: user.id },
ACCESS_TOKEN_SECRET,
{ expiresIn: '15m' }
);
// 長期間のリフレッシュトークン(7日間)
const refreshToken = jwt.sign(
{ userId: user.id },
REFRESH_TOKEN_SECRET,
{ expiresIn: '7d' }
);
// リフレッシュトークンをデータベースに保存
storeRefreshToken(user.id, refreshToken);
// アクセストークンをHTTPOnly Cookieとして設定
res.cookie('access_token', accessToken, {
httpOnly: true,
secure: true,
maxAge: 15 * 60 * 1000 // 15分
});
// リフレッシュトークンをHTTPOnly Cookieとして設定
res.cookie('refresh_token', refreshToken, {
httpOnly: true,
secure: true,
path: '/api/refresh', // リフレッシュエンドポイントのみで使用
maxAge: 7 * 24 * 60 * 60 * 1000 // 7日間
});
res.json({ success: true });
});
// トークンリフレッシュエンドポイント
app.post('/api/refresh', (req, res) => {
const refreshToken = req.cookies.refresh_token;
if (!refreshToken) {
return res.status(401).json({ error: 'リフレッシュトークンがありません' });
}
try {
// リフレッシュトークンの検証
const payload = jwt.verify(refreshToken, REFRESH_TOKEN_SECRET);
// データベースに保存されているトークンと比較
const storedToken = getStoredRefreshToken(payload.userId);
if (refreshToken !== storedToken) {
return res.status(401).json({ error: '無効なリフレッシュトークン' });
}
// 新しいアクセストークンの発行
const newAccessToken = jwt.sign(
{ userId: payload.userId },
ACCESS_TOKEN_SECRET,
{ expiresIn: '15m' }
);
// 新しいアクセストークンをCookieに設定
res.cookie('access_token', newAccessToken, {
httpOnly: true,
secure: true,
maxAge: 15 * 60 * 1000
});
res.json({ success: true });
} catch (error) {
return res.status(401).json({ error: 'リフレッシュトークンの検証に失敗' });
}
});
このリフレッシュトークンパターンは、アクセストークンの有効期限を短くすることでセキュリティリスクを低減しながら、ユーザー体験を維持できる優れた方法です。
これらのテクニックを組み合わせることで、SSRアプリケーションでの認証をより安全に実装することができます。次のセクションでは、実際の開発中によく遭遇するエラーとその解決法を見ていきましょう。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
トラブルシューティング:よくあるエラーと解決法
SSRアプリケーションでのCookieベース認証実装時には、様々なエラーに遭遇することがあります。この最終セクションでは、よくあるエラーとその解決法を具体的に解説します。
1. Cookieが送信されない問題
症状: APIリクエストにCookieが含まれていない、または認証状態が維持されない。
考えられる原因と解決策:
credentials
オプションの不足
// 問題のあるコード(Cookieが送信されない)
fetch('https://api.example.com/user');
// 修正例(Cookieを含める)
fetch('https://api.example.com/user', {
credentials: 'include'
});
SameSite属性の制限
// 問題のあるコード(クロスサイトでCookieが送信されない)
res.cookie('auth_token', token, {
httpOnly: true,
secure: true,
sameSite: 'strict' // クロスサイトリクエストでCookieが送信されない
});
// 修正例(クロスサイトでもCookieを送信)
res.cookie('auth_token', token, {
httpOnly: true,
secure: true, // HTTPSが必須
sameSite: 'none' // クロスサイトリクエストでもCookieを送信
});
HTTPSとsecure属性の不一致
// 問題のあるコード(開発環境ではHTTPなのにsecure: trueを指定)
res.cookie('auth_token', token, {
httpOnly: true,
secure: true, // HTTP接続ではCookieが送信されない
sameSite: 'lax'
});
// 修正例(環境に応じて設定を変更)
res.cookie('auth_token', token, {
httpOnly: true,
secure: process.env.NODE_ENV === 'production', // 開発環境ではfalse
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax'
});
2. CORS関連のエラー
症状: ブラウザのコンソールにCORS関連のエラーが表示され、APIリクエストが失敗する。
考えられる原因と解決策:
不適切なCORS設定
// 問題のあるコード(オリジンが一致しない)
app.use(cors({
origin: 'https://another-domain.com', // フロントエンドのオリジンと異なる
credentials: true
}));
// 修正例(正しいオリジンを指定)
app.use(cors({
origin: 'https://your-frontend-domain.com', // フロントエンドと同じオリジン
credentials: true
}));
'Access-Control-Allow-Origin' に関するエラー
Access to fetch at 'https://api.example.com/user' from origin 'https://frontend.example.com' has been blocked by CORS policy: The value of the 'Access-Control-Allow-Origin' header in the response must not be the wildcard '*' when the request's credentials mode is 'include'.
解決策:
// 問題のあるコード(ワイルドカードと認証情報を併用)
app.use(cors({
origin: '*',
credentials: true // これは併用できない
}));
// 修正例(明示的にオリジンを指定)
app.use(cors({
origin: 'https://frontend.example.com',
credentials: true
}));
// 複数オリジン対応の修正例
const allowedOrigins = ['https://frontend.example.com', 'https://admin.example.com'];
app.use(cors({
origin: function(origin, callback) {
if (!origin || allowedOrigins.indexOf(origin) !== -1) {
callback(null, true);
} else {
callback(new Error('CORS policy violation'));
}
},
credentials: true
}));
3. getServerSidePropsでのCookie取得の問題
症状: Next.jsのgetServerSideProps内でCookieを使ったAPIリクエストが失敗する。
考えられる原因と解決策:
Cookieヘッダーの不足
// 問題のあるコード(Cookieヘッダーがない)
export async function getServerSideProps(context) {
const response = await fetch('https://api.example.com/user');
// ...
}
// 修正例(Cookieヘッダーを設定)
export async function getServerSideProps(context) {
const { req } = context;
const response = await fetch('https://api.example.com/user', {
headers: {
Cookie: req.headers.cookie || ''
}
});
// ...
}
fetch APIの制限
サーバーサイドのfetchではcredentials: 'include'
が機能しないため、明示的にCookieヘッダーを設定する必要があります。
// 修正例(サーバーサイドではnodeFetchを使う方法も)
import nodeFetch from 'node-fetch';
export async function getServerSideProps(context) {
const { req } = context;
const response = await nodeFetch('https://api.example.com/user', {
headers: {
Cookie: req.headers.cookie || ''
}
});
// ...
}
4. JWT検証の失敗
症状: JWTの検証が失敗し、認証エラーが発生する。
考えられる原因と解決策:
シークレットキーの不一致
// 問題のあるコード(環境によってシークレットキーが異なる)
// 開発環境
const JWT_SECRET = 'development-secret';
// 本番環境
const JWT_SECRET = 'production-secret';
// 修正例(環境変数を使う)
const JWT_SECRET = process.env.JWT_SECRET || 'fallback-secret-key';
トークンの期限切れ
// 問題のあるコード(短すぎる有効期限)
const token = jwt.sign(
{ userId: user.id },
JWT_SECRET,
{ expiresIn: '5m' } // 5分は短すぎる場合がある
);
// 修正例(適切な有効期限)
const token = jwt.sign(
{ userId: user.id },
JWT_SECRET,
{ expiresIn: '1h' } // 1時間
);
// リフレッシュトークンパターンを使った修正例
const accessToken = jwt.sign(
{ userId: user.id },
ACCESS_TOKEN_SECRET,
{ expiresIn: '15m' }
);
const refreshToken = jwt.sign(
{ userId: user.id },
REFRESH_TOKEN_SECRET,
{ expiresIn: '7d' }
);
5. ハイドレーションエラー
症状: クライアントサイドでのハイドレーション時に警告やエラーが発生する。
Warning: Text content did not match. Server: "ようこそ、ゲストさん" Client: "ようこそ、ユーザーさん"
考えられる原因と解決策:
サーバーとクライアントでの状態の不一致
// 問題のあるコード(サーバーとクライアントで異なる状態)
function ProfilePage({ serverSideUser }) {
const [user, setUser] = useState(null); // 初期値がサーバーと異なる
useEffect(() => {
// クライアントサイドで認証状態を取得
async function fetchUser() {
const response = await fetch('/api/user', {
credentials: 'include'
});
const data = await response.json();
setUser(data.user);
}
fetchUser();
}, []);
return <div>ようこそ、{user?.name || 'ゲスト'}さん</div>;
}
// 修正例(初期状態をサーバーと一致させる)
function ProfilePage({ serverSideUser }) {
const [user, setUser] = useState(serverSideUser); // サーバーから取得した値で初期化
useEffect(() => {
// 必要に応じてクライアントサイドで更新
async function fetchUser() {
const response = await fetch('/api/user', {
credentials: 'include'
});
const data = await response.json();
setUser(data.user);
}
fetchUser();
}, []);
return <div>ようこそ、{user?.name || 'ゲスト'}さん</div>;
}
条件付きレンダリングの問題
// 問題のあるコード(サーバーとクライアントで条件が異なる可能性)
function Dashboard({ user }) {
const [isAuthenticated, setIsAuthenticated] = useState(!!user);
// クライアントサイドで条件が変わる可能性
return (
<div>
{isAuthenticated ? (
<p>認証済みコンテンツ</p>
) : (
<p>未認証コンテンツ</p>
)}
</div>
);
}
// 修正例(ハイドレーション完了後に異なるコンテンツを表示)
function Dashboard({ user }) {
const [isAuthenticated, setIsAuthenticated] = useState(!!user);
const [hydrated, setHydrated] = useState(false);
useEffect(() => {
setHydrated(true);
}, []);
// サーバーサイドとクライアントサイドで同じ初期レンダリング
if (!hydrated) {
return <div>{user ? <p>認証済みコンテンツ</p> : <p>未認証コンテンツ</p>}</div>;
}
// ハイドレーション後の条件付きレンダリング
return (
<div>
{isAuthenticated ? (
<p>認証済みコンテンツ(クライアントサイド)</p>
) : (
<p>未認証コンテンツ(クライアントサイド)</p>
)}
</div>
);
}
6. セキュリティ関連の問題
症状: セキュリティに関する警告が表示される、またはCookieが適切に保護されていない。
考えられる原因と解決策:
HTTPOnlyとSecure属性の欠落
// 問題のあるコード(セキュリティ属性がない)
res.cookie('auth_token', token, {
maxAge: 3600000 // セキュリティ属性がない
});
// 修正例(セキュリティ属性を追加)
res.cookie('auth_token', token, {
httpOnly: true, // JavaScriptからのアクセスを防止
secure: process.env.NODE_ENV === 'production', // HTTPS経由でのみ送信
sameSite: process.env.NODE_ENV === 'production' ? 'none' : 'lax',
maxAge: 3600000
});
CSRF対策の欠如
// 修正例(CSRF保護を追加)
const csrf = require('csurf');
const csrfProtection = csrf({ cookie: true });
// 保護が必要なルートでCSRF対策を適用
app.post('/api/change-password', csrfProtection, (req, res) => {
// ...
});
// フロントエンドにトークンを提供
app.get('/api/csrf-token', csrfProtection, (req, res) => {
res.json({ csrfToken: req.csrfToken() });
});
7. 環境ごとの設定ミスマッチ
症状: 開発環境では動作するが、本番環境では認証が機能しない。
考えられる原因と解決策:
環境変数の不適切な設定
// 問題のあるコード(ハードコードされた値)
const API_URL = 'http://localhost:4000';
// 修正例(環境変数を使用)
const API_URL = process.env.NEXT_PUBLIC_API_URL || 'http://localhost:4000';
環境ごとの設定ファイル
# .env.development
NEXT_PUBLIC_API_URL=http://localhost:4000
COOKIE_SECURE=false
COOKIE_SAME_SITE=lax
# .env.production
NEXT_PUBLIC_API_URL=https://api.your-domain.com
COOKIE_SECURE=true
COOKIE_SAME_SITE=none
これらの設定をコードで使用:
// 環境に応じた設定を使用
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
const cookieOptions = {
httpOnly: true,
secure: process.env.COOKIE_SECURE === 'true',
sameSite: process.env.COOKIE_SAME_SITE
};
デバッグのためのヒント
Cookieの状態を確認する
// サーバーサイドでCookieをログ出力
console.log('Server cookies:', req.cookies);
// ブラウザでCookieを確認(開発ツール > Application > Cookies)
ネットワークリクエストを監視する
ブラウザの開発者ツールでネットワークタブを使用して、以下を確認します:
- Cookieヘッダーが正しく送信されているか
- Set-Cookieヘッダーが正しく返されているか
- CORSヘッダーが正しく設定されているか
トークンの内容をデバッグする
// JWTトークンの内容をデバッグ
try {
const decoded = jwt.verify(token, JWT_SECRET);
console.log('Decoded token:', decoded);
} catch (error) {
console.error('Token verification failed:', error.message);
}
最後に
SSRアプリケーションでCookieベースの認証を実装する際には、クライアントサイドとサーバーサイドの両方での適切な設定が必要です。特に、以下の点に注意してください:
- Cookieの属性を適切に設定する: httpOnly, secure, sameSiteなど
- 環境の違いに対応する: 開発環境と本番環境で異なる設定を使用
- CORSを正しく設定する: credentialsオプションとoriginの設定に注意
- セキュリティ対策を十分に行う: CSRF対策やXSS対策を実装
- デバッグ情報を活用する: エラーメッセージやネットワークリクエストを確認
これらの点に注意して実装することで、安全で信頼性の高いCookieベースの認証システムを構築することができます。
適切な実装によって、ユーザー体験を損なうことなく、セキュリティを確保したSSRアプリケーションを開発しましょう。
このトピックはこちらの書籍で勉強するのがおすすめ!
この記事の内容をさらに深く理解したい方におすすめの一冊です。実践的な知識を身につけたい方は、ぜひチェックしてみてください!
おすすめコンテンツ
おすすめNode.js2025/5/20Node.jsアプリケーションのCORSエラー解決法:5分で実装できる完全ガイド
フロントエンドとバックエンドの連携で頻繁に遭遇するCORSエラーの原因と解決策を具体的なコード例とともに解説します。Node.js環境でのCORSポリシーの正しい設定方法から、開発環境と本番環境での違...
続きを読む React2025/5/1Next.jsとTypeScriptで作る高速SSGブログ:初心者でも簡単に実装できる完全ガイド
Next.jsとTypeScriptを組み合わせたSSGブログの作り方を解説します。静的サイト生成の利点から実装方法、デプロイまで、初心者でも理解できるようステップバイステップで説明。コードサンプル付...
続きを読む Docker2025/5/20Dockerコンテナ内Node.jsアプリの環境変数トラブル解決法
Dockerコンテナ内でNode.jsアプリケーションを実行すると、環境変数が正しく読み込まれない問題に遭遇することがあります。この記事では、具体的な原因と解決策を実用的なコード例で解説します。
続きを読む JavaScript2025/5/20Express.jsのmiddlewareでasync/await関数が動かない時の解決法
Express.jsでasync/awaitを使用したミドルウェア関数でエラーハンドリングができない問題の解決策を紹介します。この頻出するバグの原因と解決方法を具体的なコード例で詳しく解説します。
続きを読む Next.js2025/5/20Next.jsのAPIルートでホットリロードが効かない時の解決法
Next.jsの開発中にAPIルートのコード変更が反映されない問題に対処する方法を紹介します。この記事では、環境設定を見直し、効率的に開発を続けるためのヒントを共有します。
続きを読む JavaScript2025/5/14WebSocketを活用したリアルタイムチャットアプリケーション開発ガイド
WebSocketを使って初心者でも作れるリアルタイムチャットアプリケーションの開発方法を解説します。基本概念から実装、デプロイまでステップバイステップで紹介します。
続きを読む JavaScript2025/5/5Node.jsとWebSocketで作る!初心者でも実装できるリアルタイムWebアプリケーション開発チュートリアル
'Webの世界は常に進化しています。かつては「リクエストを送信して応答を待つ」という単方向の通信が一般的でしたが、現代のユーザーはよりダイナミックな体験を求めています。リアルタイムWebアプリケーショ...
続きを読むGo
2025/5/2Golangの並行処理パターン:効率的なアプリケーション開発のための実践ガイド
Golangのgoroutineとチャネルを使った並行処理パターンを実践的なコード例とともに解説。基本から応用まで、効率的な非同期処理の実装方法とよくあるエラーの回避策を紹介します。
続きを読む